If you have a few years of experience in the DevOps ecosystem, and you're interested in sharing that experience with the community, have a look at our Contribution Guidelines.
How to Check if a Pipeline Parameter Is Empty in Jenkins
Last updated: May 12, 2024
1. Overview
Jenkins parameters provide a way to input data into our pipeline and dynamically control its flow. For example, we can decide which environment we want the pipeline to deploy to depending on a parameter value of prod, staging, or testing.
We can also use a shared pipeline code and provide dynamic parameters to deploy multiple services or applications. This comes in handy to reduce the repetition and make it easier to apply changes in a shared location.
In this tutorial, we’re going to show how we can define and use a parameter in Jenkins. Then, we’ll look at different ways that we can check if this parameter is empty.
2. Defining Parameters in Jenkins
There are multiple locations where we can define Jenkins parameters in a pipeline job. We can create them from the pipeline configuration GUI or from inside the Jenkinsfile itself.
2.1. Defining Parameters in the Pipeline Configuration
The easiest way to define a Jenkins parameter is through the GUI. We can simply go to the pipeline configuration for our pipeline and select the checkbox for This project is parameterized:
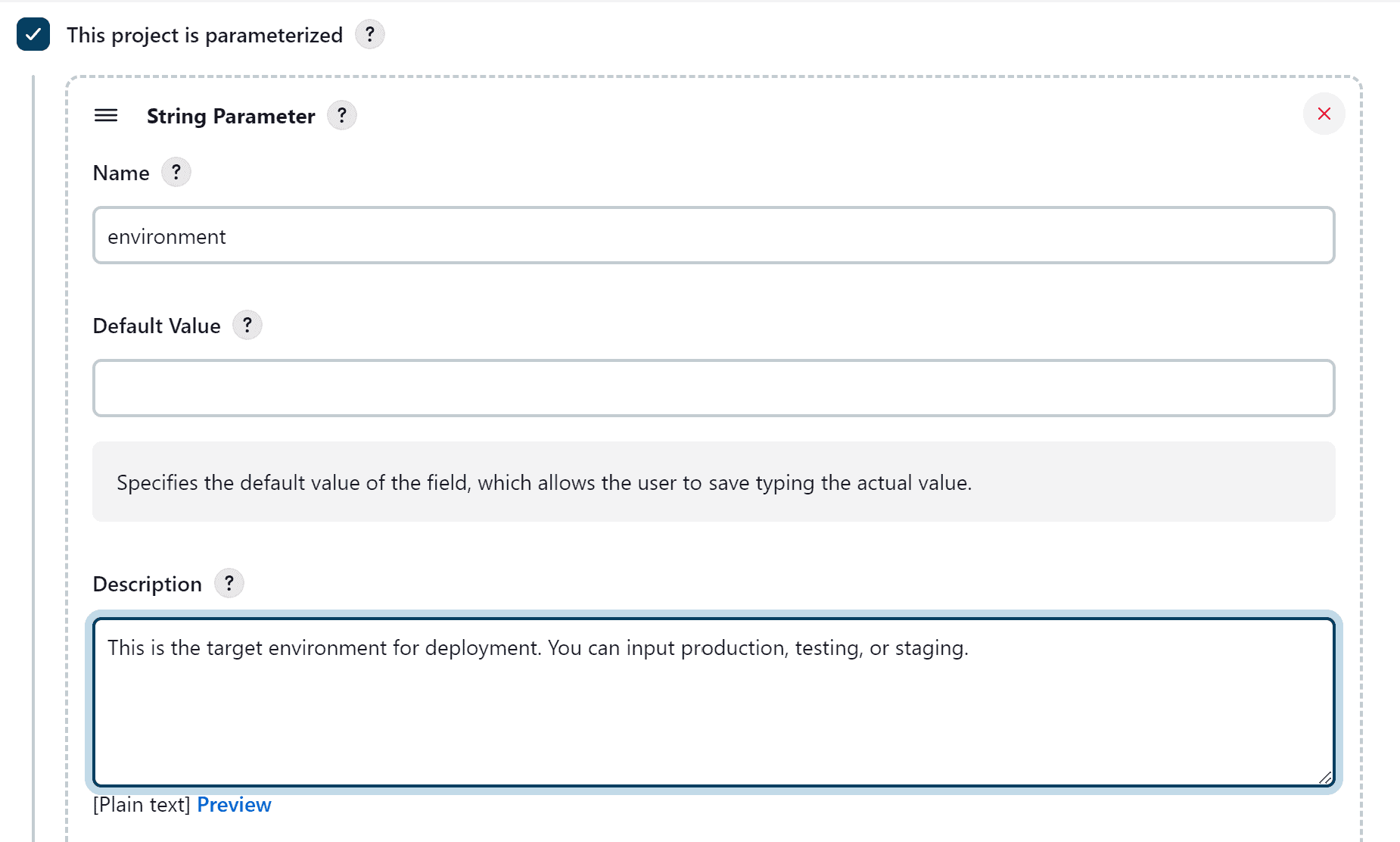
Here we’ve created a new parameter with the name environment and of type String. We provided a description to make it clear to a user what input is expected for this parameter.
2.2. Defining Parameters in the Jenkinsfile
The other way to define parameters is by specifying them in the Jenkinsfile. To do this, we use a parameters directive under the pipeline block:
pipeline {
agent any
parameters {
string description: 'This is the target environment for deployment', name: 'environment'
}
}
In this example, we defined the parameter the same as we did in the GUI, but in the script form. Of course, we can only create a parameter in one of the two ways, but not both.
3. Printing the Parameters Value
Now that we’ve defined our parameter, let’s use it simply by printing its value in our pipeline:
pipeline {
agent any
parameters {
string description: 'This is the target environment for deployment', name: 'environment'
}
stages {
stage("print parameter value"){
steps {
echo "we'll deploy on the $environment environment"
}
}
}
}
Above, we used the echo step to print our parameter as part of a message output. Now let’s trigger our pipeline and see the result:
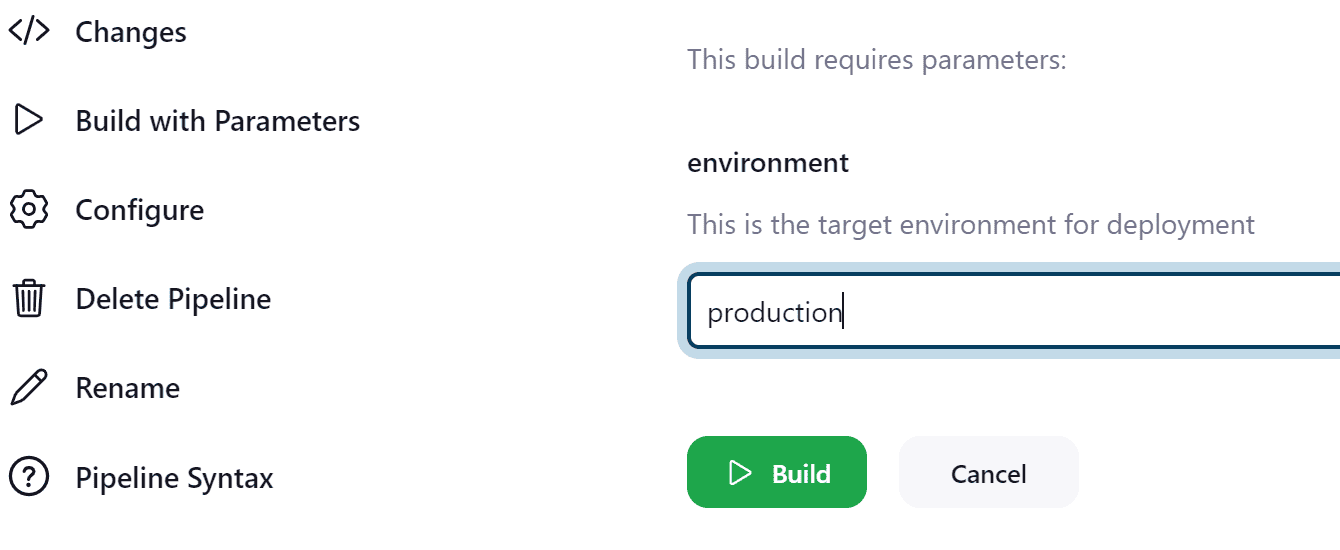
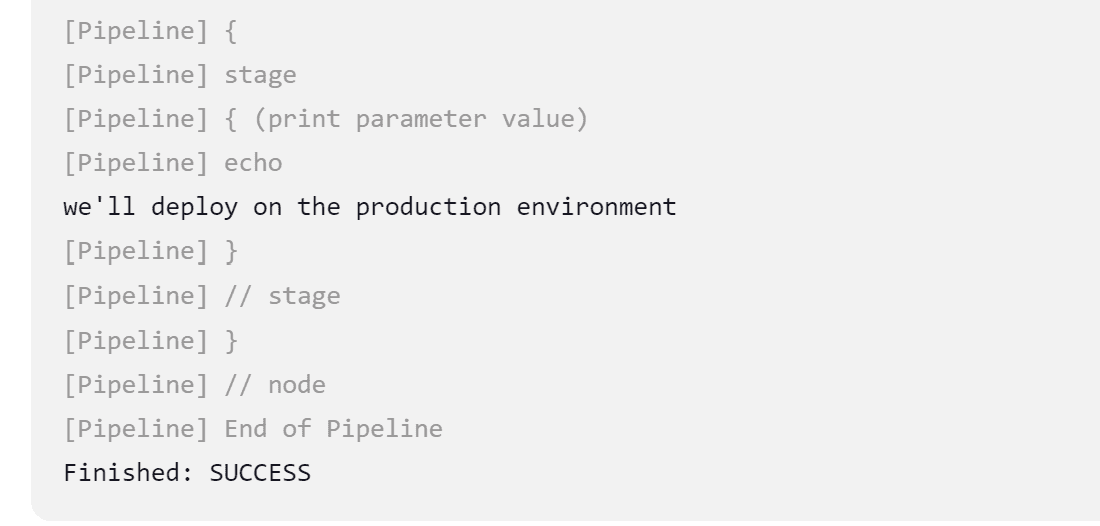
As we can see, we provided the input production for our parameter, and it was printed in the pipeline console output.
4. Checking if a Jenkins Parameter Is Empty
When a parameter value is empty, it can cause unexpected behavior in the pipeline flow. So, it’s a good practice to handle empty parameters and apply a proper action whether by throwing an error or setting a default value.
Now let’s see how we can handle an empty parameter in Jenkins.
4.1. Using the isEmpty() Function
The simplest way to check an empty parameter is to use the isEmpty() function. It returns a boolean value of true when the string is empty and false if it contains any characters.
Let’s check this on our previous parameter:
pipeline {
agent any
parameters {
string description: 'This is the target environment for deployment', name: 'environment'
}
stages {
stage("print parameter value") {
steps {
script {
if (environment.isEmpty()) {
echo "The environment parameter cannot be empty"
}
else {
echo "we'll deploy on the $environment environment"
}
}
}
}
}
}
Here we’re using the isEmpty() function inside an if condition to check our environment parameter. It’s important to note that using if-else statements in the pipeline requires adding them inside a script block. This is because if-else statements are part of the Groovy script and not a Jenkins step.
Now let’s trigger our pipeline with an empty parameter:
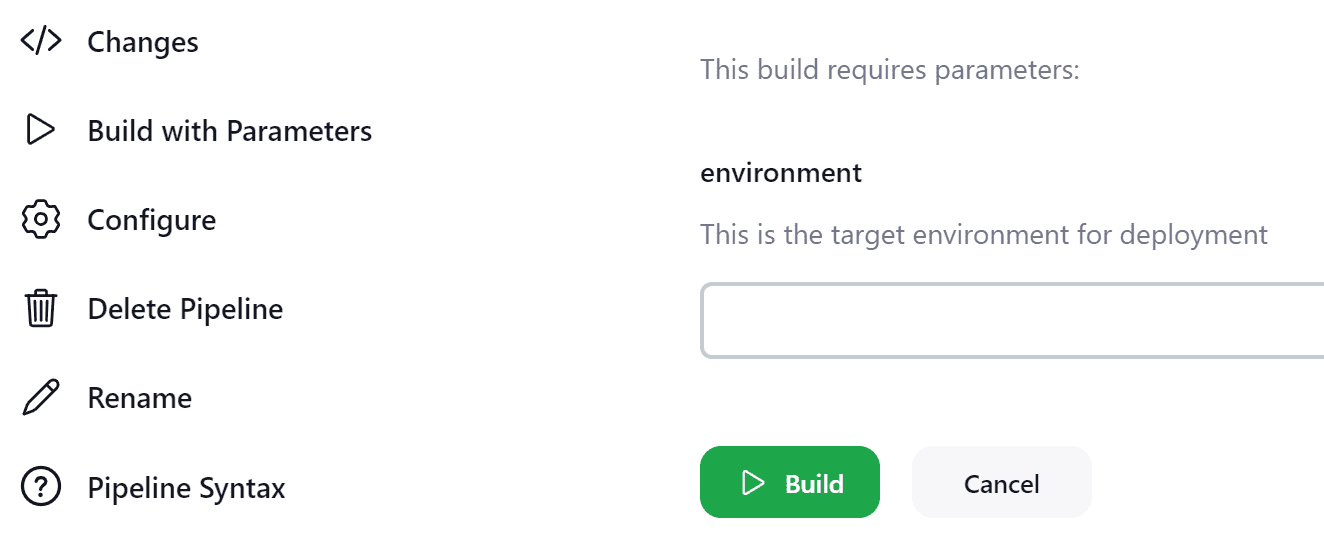
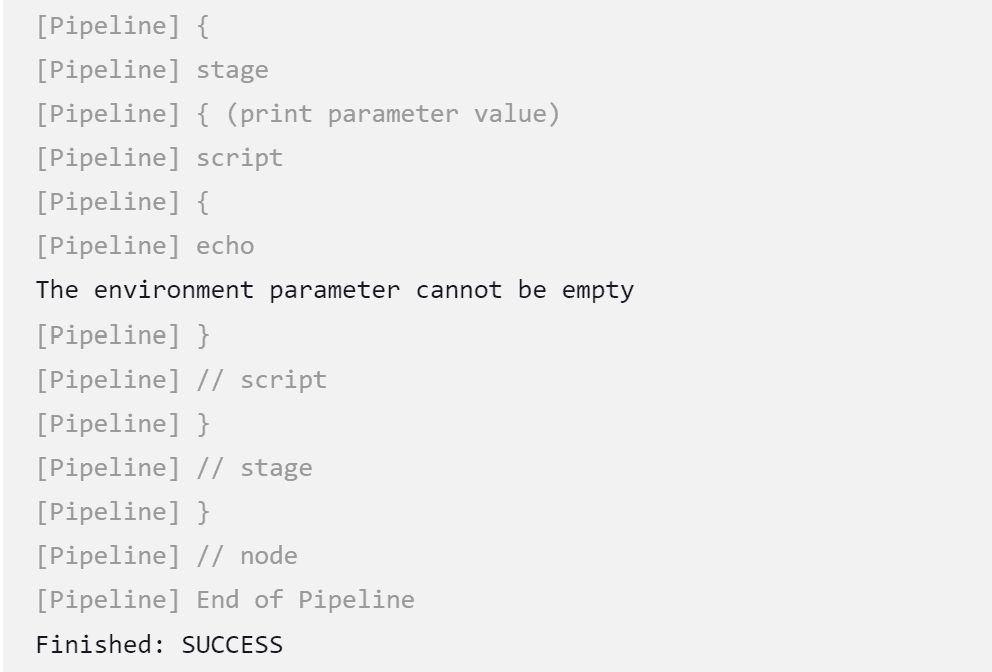
We can see that the output message we’ve set in our pipeline shows up when the parameter is empty.
4.2. Using if-else Statement
Another way of checking an empty parameter is to compare it directly with an empty string or null using an if-else statement. So, we can simply replace our previous function with the conditional statement:
pipeline {
agent any
parameters {
string description: 'This is the target environment for deployment', name: 'environment'
}
stages {
stage("print parameter value") {
steps {
script {
if (environment == '' || environment == null) {
echo "The environment parameter is again empty"
}
else {
echo "we'll deploy on the $environment environment"
}
}
}
}
}
}
Here we just replaced the isEmpty() function with sort of a raw if-else statement. We’ve modified our output message a little to differentiate the output from the previous scenario.
Let’s trigger our pipeline again, leaving the parameter empty:
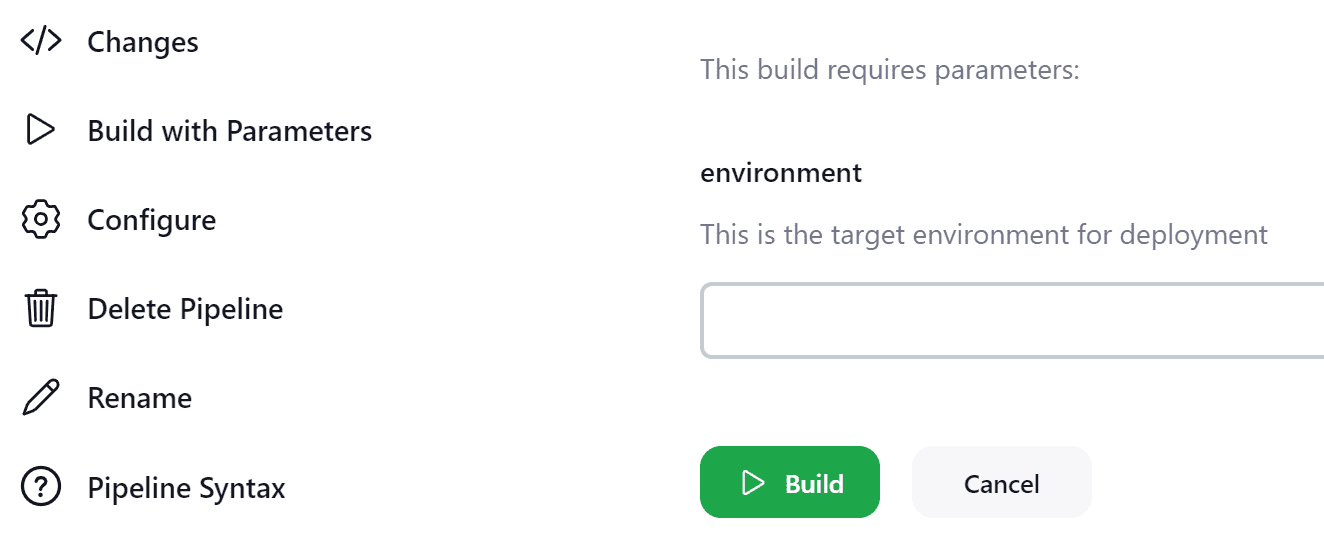
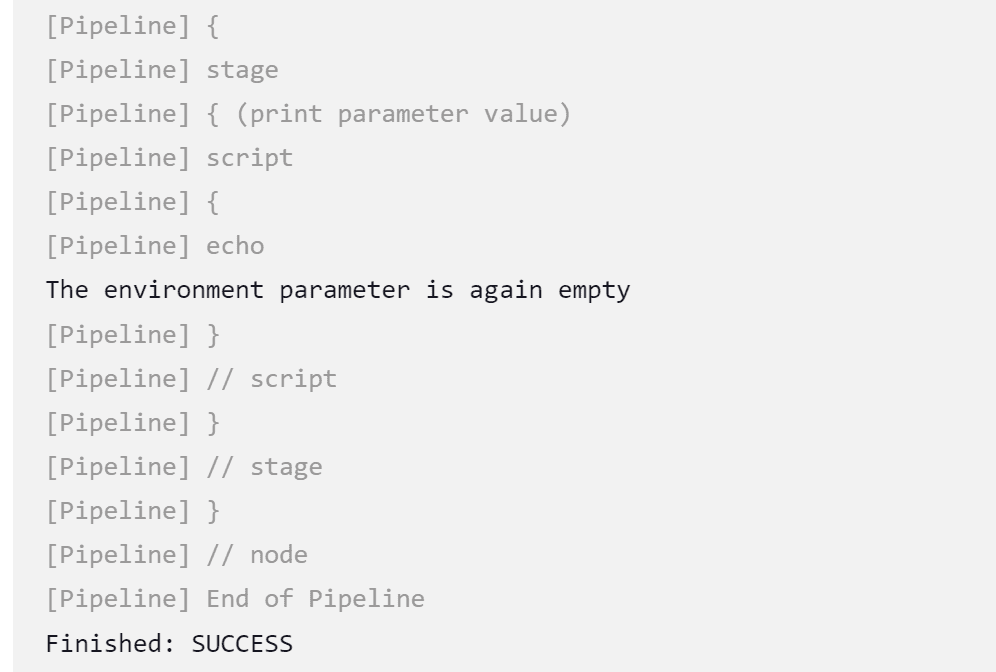
As seen above, the output message is displayed when we provide an empty value for our parameter.
5. Conclusion
In this article, we’ve covered the basics of Jenkins parameters and how to check if a parameter value is empty. We explained different ways of defining parameters using the pipeline configuration and the declarative pipeline script in the Jenkinsfile. We also showed how to use the isEmpty() string function or an if-else statement to check if a parameter is empty.